Resize Image Javascript in Input Before Upload
Epitome resizing is computationally expensive and usually done on the server-side and so that right-sized paradigm files are delivered to the client-side. This arroyo also saves data while transmitting images from the server to the client.
Notwithstanding, in that location are a couple of situations where y'all might demand to resize images purely using JavaScript on the client side. For instance -
- Resizing images before uploading to server
Uploading a large file on your server volition have a lot of time. You can first resize images on the browser and so upload them to reduce upload time and improve application performance.
- Rich prototype editors that work on client-side
A rich epitome editor that offers image resize, crop, rotation, zoom IN and zoom OUT capabilities ofttimes require image manipulation on the client-side. The speed is critical for the user in these editors.
If a user is manipulating a heavy image, it will take a lot of fourth dimension to download transformed images from the server. Imagine this with operations like undo/redo and complex text and image overlays.
Image manipulation in JavaScript is done using the canvas element. There are libraries like fabric.js that offer rich APIs.
Apart from the above 2 reasons, in almost all cases, you would want to get the resized images from the backend itself and then that client doesn't have to deal with heavy processing tasks.
In this post-
- We will offset talk about how to practice resizing purely in JavaScript using the
sheet
element.
- Then we volition comprehend in slap-up detail how you can resize, crop, and practise a lot with images by changing the epitome URL in the
src
aspect. This is the preferred way to resize images without degrading the user experience programmatically.Besides, we volition learn how y'all tin can practise this without needing to set upward any libraries or backend servers.
Prototype resizing in JavaScript - Using sail element
The HTML <sheet>
chemical element is used to draw graphics, on the wing, via JavaScript. Resizing images in browser using canvas
is relatively uncomplicated.
drawImage
office allows u.s.a. to render and scale images on canvas element.
drawImage(image, x, y, width, height)
The first statement image
tin can be created using the Image()
constructor, as well equally using any existing <img>
element.
Permit's write the code to resize a user-uploaded epitome on the browser side 300x300
.
<html> <body> <div> <input type="file" id="image-input" have="image/*"> <img id="preview"></img> </div> <script> let imgInput = document.getElementById('image-input'); imgInput.addEventListener('change', function (e) { if (e.target.files) { permit imageFile = due east.target.files[0]; var reader = new FileReader(); reader.onload = role (e) { var img = certificate.createElement("img"); img.onload = part (event) { // Dynamically create a canvas chemical element var sail = document.createElement("canvas"); // var canvas = certificate.getElementById("sheet"); var ctx = canvas.getContext("2nd"); // Actual resizing ctx.drawImage(img, 0, 0, 300, 300); // Show resized image in preview chemical element var dataurl = canvas.toDataURL(imageFile.type); certificate.getElementById("preview").src = dataurl; } img.src = e.target.outcome; } reader.readAsDataURL(imageFile); } }); </script> </trunk> </html>
Allow's understand this in parts. Get-go, the input file type field in HTML
<html> <body> <div> <input type="file" id="image-input" accept = "paradigm/*"> <img id="preview"></img> </div> </body> </html>
At present we need to read the uploaded image and create an img
element using Image()
constructor.
let imgInput = certificate.getElementById('epitome-input'); imgInput.addEventListener('alter', function (e) { if (e.target.files) { let imageFile = e.target.files[0]; var reader = new FileReader(); reader.onload = function (e) { var img = document.createElement("img"); img.onload = function(event) { // Actual resizing } img.src = e.target.result; } reader.readAsDataURL(imageFile); } });
Finally, let's draw the image on sail and show preview element.
// Dynamically create a canvas chemical element var canvas = document.createElement("canvas"); var ctx = canvas.getContext("2d"); // Bodily resizing ctx.drawImage(img, 0, 0, 300, 300); // Show resized image in preview chemical element var dataurl = canvas.toDataURL(imageFile.type); document.getElementById("preview").src = dataurl;
You might notice that the resized image looks distorted in a few cases. It is because we are forced 300x300
dimensions. Instead, we should ideally only manipulate one dimension, i.e., height or width, and accommodate the other accordingly.
All this tin be done in JavaScript, since you have admission to input paradigm original elevation (img.width
) and width using (img.width
).
For example, we can fit the output image in a container of 300x300
dimension.
var MAX_WIDTH = 300; var MAX_HEIGHT = 300; var width = img.width; var acme = img.acme; // Change the resizing logic if (width > height) { if (width > MAX_WIDTH) { top = height * (MAX_WIDTH / width); width = MAX_WIDTH; } } else { if (height > MAX_HEIGHT) { width = width * (MAX_HEIGHT / elevation); superlative = MAX_HEIGHT; } } var canvas = document.createElement("sail"); canvas.width = width; sail.meridian = pinnacle; var ctx = canvas.getContext("2d"); ctx.drawImage(img, 0, 0, width, height);
Controlling epitome scaling behavior
Scaling images tin result in fuzzy or blocky artifacts. There is a trade-off between speed and quality. By default browsers are tuned for better speed and provides minimum configuration options.
Y'all can play with the following backdrop to control smoothing result:
ctx.mozImageSmoothingEnabled = false; ctx.webkitImageSmoothingEnabled = false; ctx.msImageSmoothingEnabled = fake; ctx.imageSmoothingEnabled = false;
Epitome resizing in JavaScript - The serverless manner
ImageKit allows you to manipulate image dimensions directly from the image URL and get the exact size or crop you want in real-fourth dimension. Start with a single chief image, every bit large as possible, and create multiple variants from the same.
For case, we can create a 400 ten 300
variant from the original epitome like this:
https://ik.imagekit.io/ikmedia/ik_ecom/shoe.jpeg?tr=westward-400,h-300
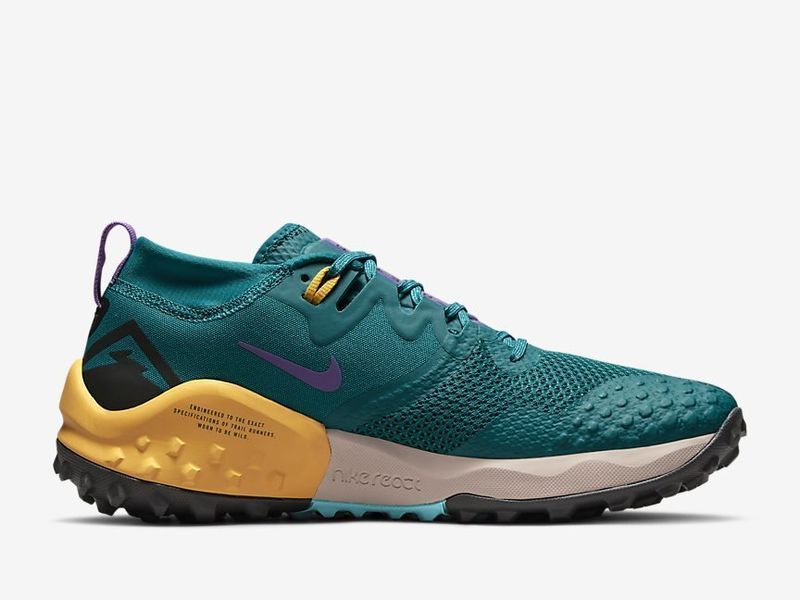
Yous can utilise this URL straight on your website or app for the product epitome, and your users get the right image instantly.
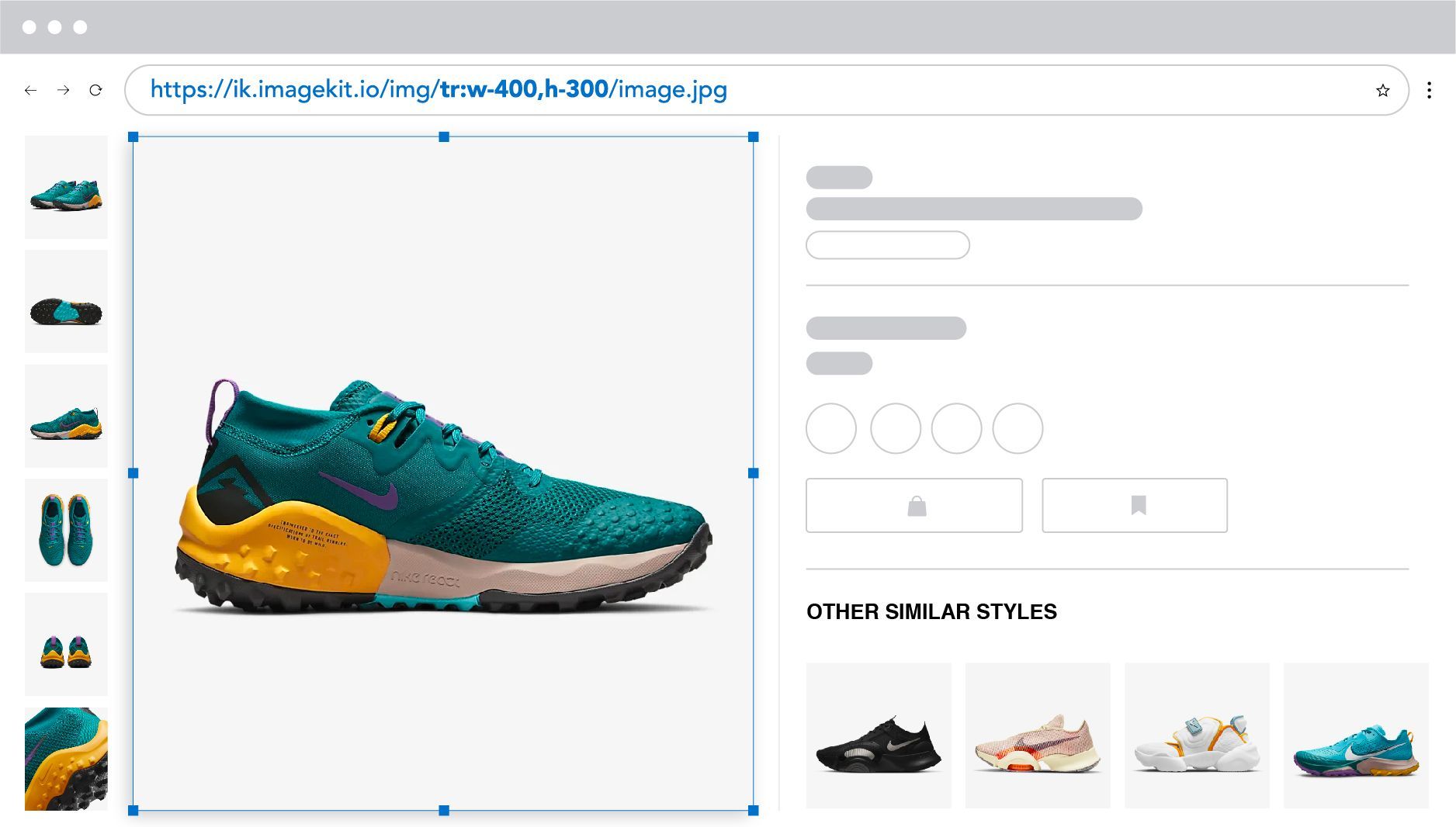
If you don't want to ingather the image while resizing, there are several possible ingather modes.
https://ik.imagekit.io/ikmedia/ik_ecom/shoe.jpeg?tr=w-400,h-300,cm-pad_resize,bg-F5F5F5
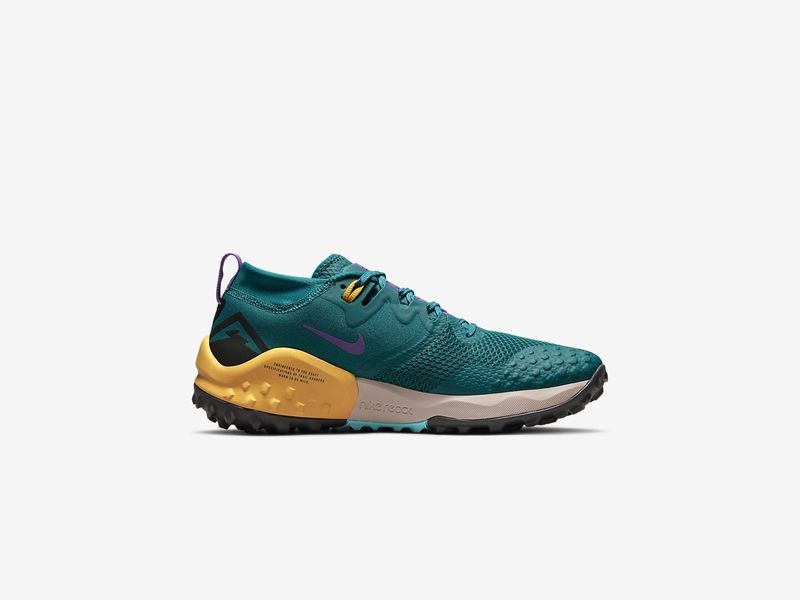
We have published guides on how y'all tin can do the following things using ImageKit's real-time image manipulation.
- Resize epitome - Basic elevation & width manipulation
- Cropping & preserving the attribute ratio
- Face and object detection
- Add a watermark
- Add a text overlay
- Adapt for slow cyberspace connection
- Loading a blurred low-quality placeholder
Summary
- In most cases, you should not exercise epitome resizing in the browser considering it is slow and results in poor quality. Instead, yous should utilise an paradigm CDN like ImageKit.io to resize images dynamically by changing the epitome URL. Try our forever gratis programme today!
- If your use-case demands client-side resizing, it is possible using the
sheet
element.
Source: https://imagekit.io/blog/how-to-resize-image-in-javascript/
0 Response to "Resize Image Javascript in Input Before Upload"
Post a Comment